Emotion CSS in JS: An Alternative To React Styled Components
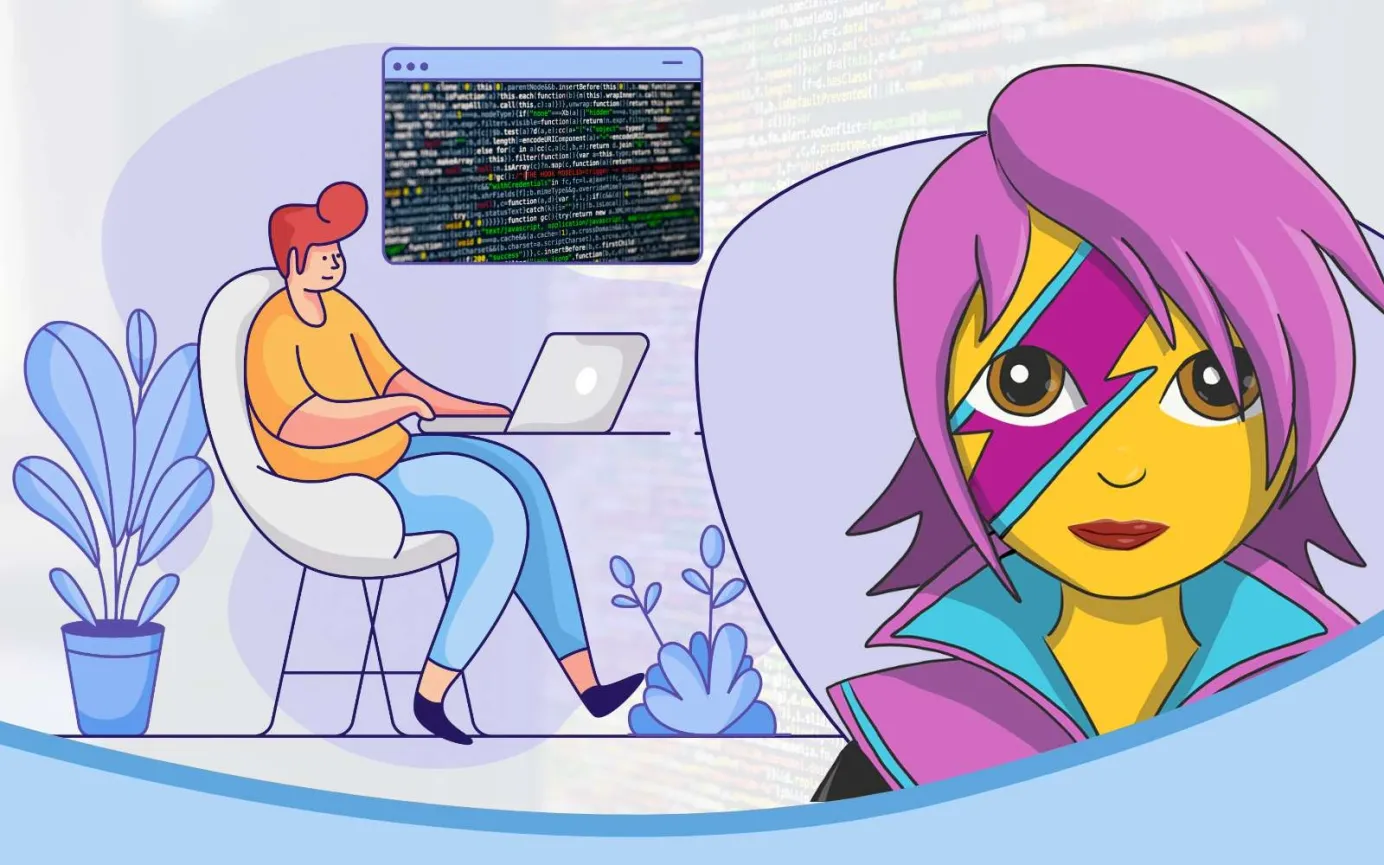
Emotion is a popular CSS in JS alternative to React Styled Components. Learn Emotion by example in this short primer for developers. No fluff, no fat, just a concise kickstart to get you coding quickly.
How To Use @emotion/styled
Emotion is a CSS in Javascript library that can be used to write cascading stylesheet (CSS) code using Javascript. Emotion provides a structured and predictable approach to writing CSS styles and includes features such as source maps, labels and testing utilities. A React version of Emotion is available (@emotion/react) as well as a so-called agnostic version (@emotion/css) which is framework independent
@emotion/styled is the styled application programming interface (API) for the React version of Emotion. @emotion/styled is similar to Styled Components, so if you have experience with Styled Components you should be able to pick up Emotion’s styled components quickly. This article will demonstrate some basic uses of @emotion/styled to get you started.
Let's start by creating a styled paragraph with an orange background.
import styled from ‘@emotion/styled’
const OrangeParagraph = styled.p`
background-color: orange;`
const MyComponent = () => {
return (
<OrangeParagraph>
This is an Orange Paragraph
</OrangeParagraph>
)
}
We can also add props to our components for even more customization like:
const Title = styled.h1`
color: ${props => props.color};
`
An alternative method of achieving the same outcome would be:
const Title = styled.h1`
color: ${({color}) => color};
`
These props can then be used like this:
const MyComponent = () => {
return (
<Title color="red">This is the title</Title>
)
}
SCSS syntax
Emotion's styled components allows for SCSS syntax. For example, we can make every span inside a p tag have the color red like this:
const Paragraph = styled.p`
span {
color: red;
}
`
Object syntax
@emotion/styled also supports object syntax so that you can use objects instead of template strings:
const Button = styled.button(
{
backgroundColor: ‘white’,
border: ‘2px solid black’
}
)
Props and child selectors work in the object syntax like this:
const Button = styled.button(
{
background: ‘white’,
‘&:hover’: {
color: ‘orange’
}
},
props => ({
color: props.color
})
)
Styling React components
We can also style our own React components as long as they accept a className prop like this:
const Section = (props) => (
<section className={props.className}>This is a section</section>
)
const PurpleSection = styled(Section)`
color: purple;
`
Copy styles between components
Another trick to make your code neater is the withComponent method which copies the styles from one component to another:
const Section = styled.section`
background: black;
color: white;
`
const Div = Section.withComponent(‘div’);
const MyComponent = () => {
return (
<Section>section</Section>
<Div>div</Div>
)
}
We can also replicate the above code using the as prop:
const MyComponent = () => {
return (
<Section>section</Section>
<Section as="div">div</Section>
)
}
Learn more
This article has covered the basics of using Emotion’s styled component system (@emotion/styled).
For further details, please refer to the official docs at: https://emotion.sh/docs/styled.
Thought Resort Free E-Zine
Sign up to receive the latest articles in your inbox.