React Virtualized: Next-Level Data Rendering
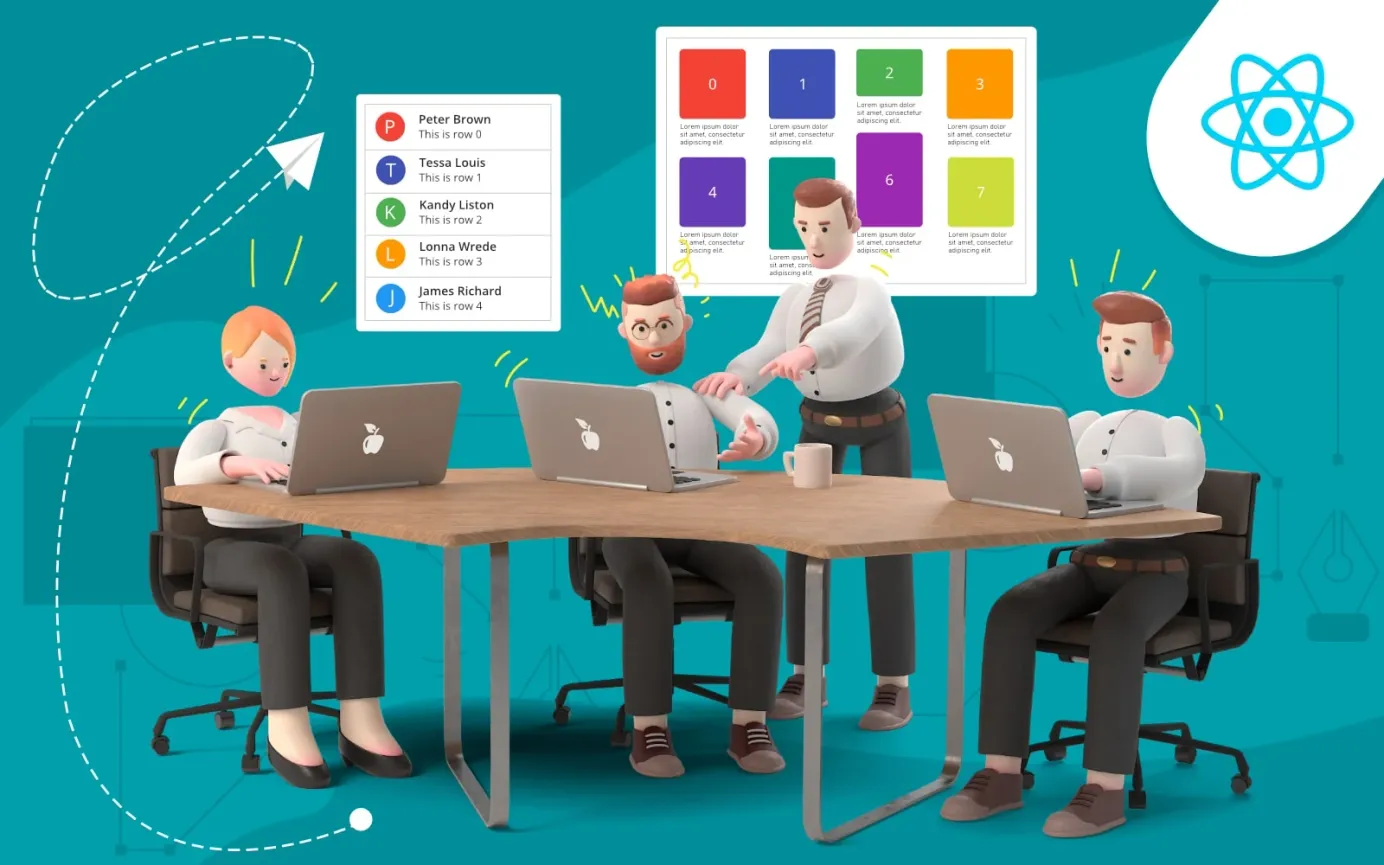
React Virtualized is a versatile and widely-acclaimed UI component library for integrating large datasets into your React apps.
React Virtualized is a widely utilized library that streamlines the rendering of long lists and large datasets with React.js. With 24.5k Github stars as of December 2022, React Virtualized is strong solution for incorporating large volumes of data into your applications without compromising user experience.
React Virtualized deploys a clever rending algorithm to render only the elements that are visible inside the viewport, and streamlines its use of DOM elements thereby optimizing app performance.
Overview of components available
React Virtualized allows developers to render a high volume of data at speed. It provides basic data components, including:
- Lists
- Tables
- Grids - to render checkboard data
- Collections - more flexible than grids, you can render data that is overlapping or arbitrarily positioned
- Masonry layouts (pictured below)
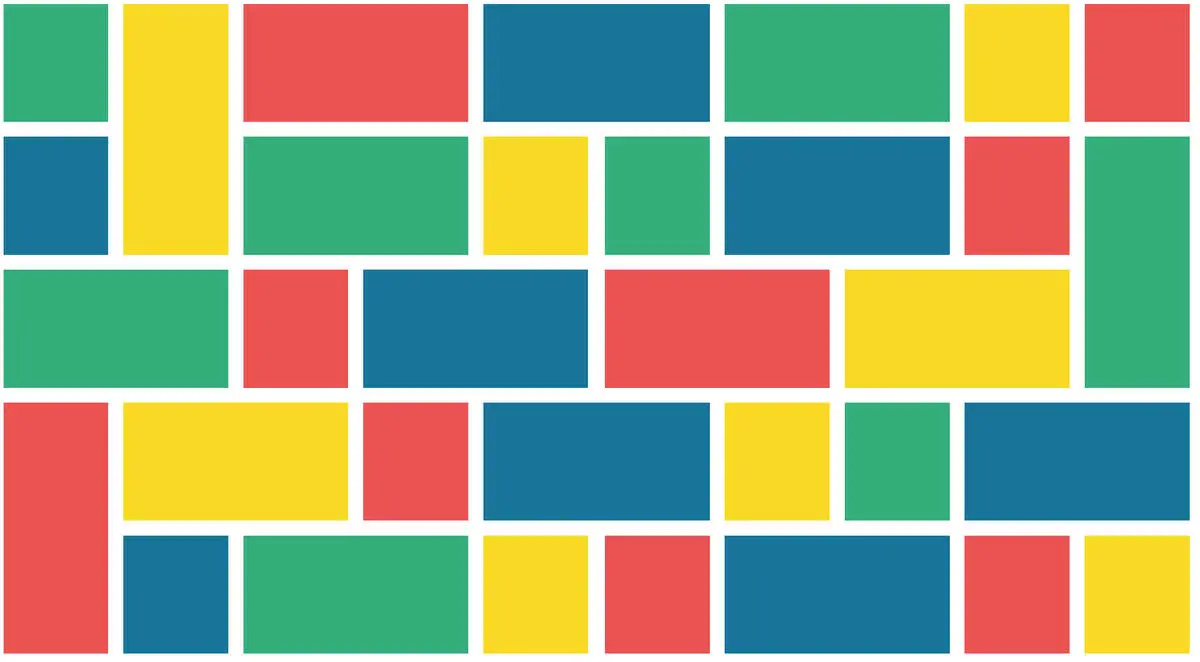
React Virtualized also includes some extremely useful higher-order components such as:
- MultiGrid - seamlessly attaches multiple grids to form a fixed column/row interface.
- ScrollSync - synchronizes the scrolling between two or more components in a manner similar to freezing a row or column on a spreadsheet.
- ArrowKeyStepper - allow your users to traverse your lists, tables and grids with their arrow keys.
- Autosizer - automatic management of the width and height of list items and table cells.
- CellMeasurer - just-in-time measurement of dynamic content
- InfiniteLoader - just-in-time data fetching to ensure data is ready just prior to your users scrolling to it.
Features of React Virtualized
Key features include:
- Grid and list components
React Virtualization offers a range of grid and list components which you can use to showcase your data. These components are created with an adjustable virtualization engine, which allows them to display expansive amounts of information without compromising performance or user experience. - Support for different data formats and layouts
Leveraging this data-agnostic library, you can showcase and manipulate data in your React applications in a variety of formats - from lists to grids, tables to trees. - Column and row resizing
With React Virtualized, you can give your users with the capability to resize columns and rows within grid and table components, allowing them to tailor the data layout to their needs and preferences. - Horizontal and vertical scrolling
React Virtualised makes it effortless for your users to traverse horizontally and vertically within your list and grid components, meaning that they can explore large amounts of data in one place. - Customizable styles and themes
Customize components with CSS and custom themes to match your brand aesthetics.
How to install React Virtualized
To install React Virtualized via npm, first verify you have both Node.js and npm installed on your system with the following shell commands, which should return the version number of each:
node -v
npm -v
Once you have confirmed that npm and Node.js are installed, change to the folder containing the project for which you are planning to use React Virtualized, then run the following command to install the package with its dependencies:
npm install react-virtualized
Alternatively, you can use the yarn package manager to quickly install it with the following shell command:
yarn add react-virtualized
Once installed, simply import React Virtualized components into your existing React code and begin using them.
React Virtualized examples
Basic example
Here's an example of how you can efficiently render a lengthy list of items using the React Virtualized library:
import React from 'react';
import { List } from 'react-virtualized';
function MyList({ items }) {
return (
<List
width={300}
height={300}
rowCount={items.length}
rowHeight={20}
rowRenderer={({ index, key, style }) => (
<div key={key} style={style}>
{items[index]}
</div>
)}
/>
);
}
In the example above, we use the React Virtualized library's List component to define the MyList function, which displays a list of items passed to it. The size and layout of the list items are controlled through props such as width, height, rowCount and rowHeight, while the rowRenderer function specifies how each list item should styled.
Other Examples
Example 1: Rendering a long list
In this example, Greg Pascale renders a long list using React Virtualized. Click on the Babel, CSS and HTML buttons to view the code.
Example 2: Creating a scrolling table using React Virtualized
CodePen user abidibo demonstrates how to use React Virtualized to create a table with many rows and a vertical scroll feature:
Interactive demos
Follow the links below to view React Virtualized in action:
More information
For further details and documentation, please visit the React Virtualized Git repo:
Thought Resort Free E-Zine
Sign up to receive the latest articles in your inbox.